Feature Test
Old Feature Test Page
yash101
Published 1/30/2025
Updated 1/30/2025
This article is a feature test - tries to test different behaviors of Markdown, code and math.
Is this section being rendered correctly? This Hero content was written with Markdown.
#
Jupyter Code Blocks
#
Cell with successful output
# Code block and output
for i in range(5):
print(f'Hello, {i}')
def hello_world():
print('hello world')
Hello, 0
Hello, 1
Hello, 2
Hello, 3
Hello, 4
#Code block with
Code block with stdout
, stderr
and an Exception
# Stdout, stderr and runtime error
import sys
for i in range(10):
if i % 2 == 0:
print(f'Hello, {i}')
else:
print(f'Hello, {i}', file=sys.stderr)
x = None
x.doSomeMethodThatDoesNotExist()
Hello, 0
Hello, 2
Hello, 4
Hello, 6
Hello, 8
Hello, 1
Hello, 3
Hello, 5
Hello, 7
Hello, 9
#
Code cell with Pyplot display and Pandas Table
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
# Generate random data for a plot
x = np.linspace(0, 10, 100)
y = np.sin(x) + np.random.normal(scale=0.1, size=len(x))
plt.figure(figsize=(8, 5))
plt.plot(x, y, label="Noisy Sine Wave", color="blue", linewidth=2)
plt.xlabel("X Axis")
plt.ylabel("Y Axis")
plt.title("Sample Matplotlib Plot")
plt.legend()
plt.grid(True)
plt.show()
# Generate a sample DataFrame
data = {
"A": np.random.randint(0, 100, 10),
"B": np.random.rand(10),
"C": np.random.choice(["Yes", "No"], 10),
}
df = pd.DataFrame(data)
# Display DataFrame
df
A | B | C | |
---|---|---|---|
0 | 42 | 0.563690 | Yes |
1 | 83 | 0.158875 | Yes |
2 | 63 | 0.461055 | Yes |
3 | 85 | 0.535424 | Yes |
4 | 59 | 0.870812 | Yes |
5 | 41 | 0.623846 | No |
6 | 20 | 0.049943 | No |
7 | 60 | 0.206974 | No |
8 | 49 | 0.563570 | No |
9 | 72 | 0.964520 | No |
#
Escaping HTML in code blocks
print('<h1>Hello world!</h1>')
print('<h1>Hello world!</h1>')
<h1>Hello world!</h1>
<h1>Hello world!</h1>
#
Markdown
Tests on Markdown features
#
Headings:
#
Top level heading (h1)
#
Heading h2
#
Heading h3
#
Heading h4
#
Heading h5
#
Heading h6
#
Bullet Points, Nested bullet points
- Bullet point 1
- Bullet point 2
- Nested bullet point 2.1
- Nested bullet point 2.1.1
- Nested bullet point 2.1.2
- Nested bullet point 2.1
- Bullet point 3
#
Numeric Lists
- Bullet point 1
- Bullet point 2
- Bullet point 2.1
- Bullet point 2.2
- Bullet point 2.2.1
- Bullet point 2.2.2
- Bullet point 3
- Bullet point 4
#
Task List (Currently unsupported)
- [x] Write the press release
- [ ] Update the website
- [ ] Contact the media
#
Code Blocks (inline and block)
Hello world, this is a code block
. Lorem ipsum dolor sit amet, consectet
ur adipiscing elit.
Code block without language description:
function extractAttachments(blob) {
const attachments = Object
.entries(blob)
.map(([identifier, attachment]) => {
const mime = Object.keys(attachment)[0];
const data = Buffer.from(attachment[mime], 'base64');
const meta = probe.sync(data);
return {
identifier,
meta,
data,
};
});
return attachments;
}
Code block with language description:
function extractAttachments(blob) {
const attachments = Object
.entries(blob)
.map(([identifier, attachment]) => {
const mime = Object.keys(attachment)[0];
const data = Buffer.from(attachment[mime], 'base64');
const meta = probe.sync(data);
return {
identifier,
meta,
data,
};
});
return attachments;
}
#
Math
Inline equation
Display math:
#
Images
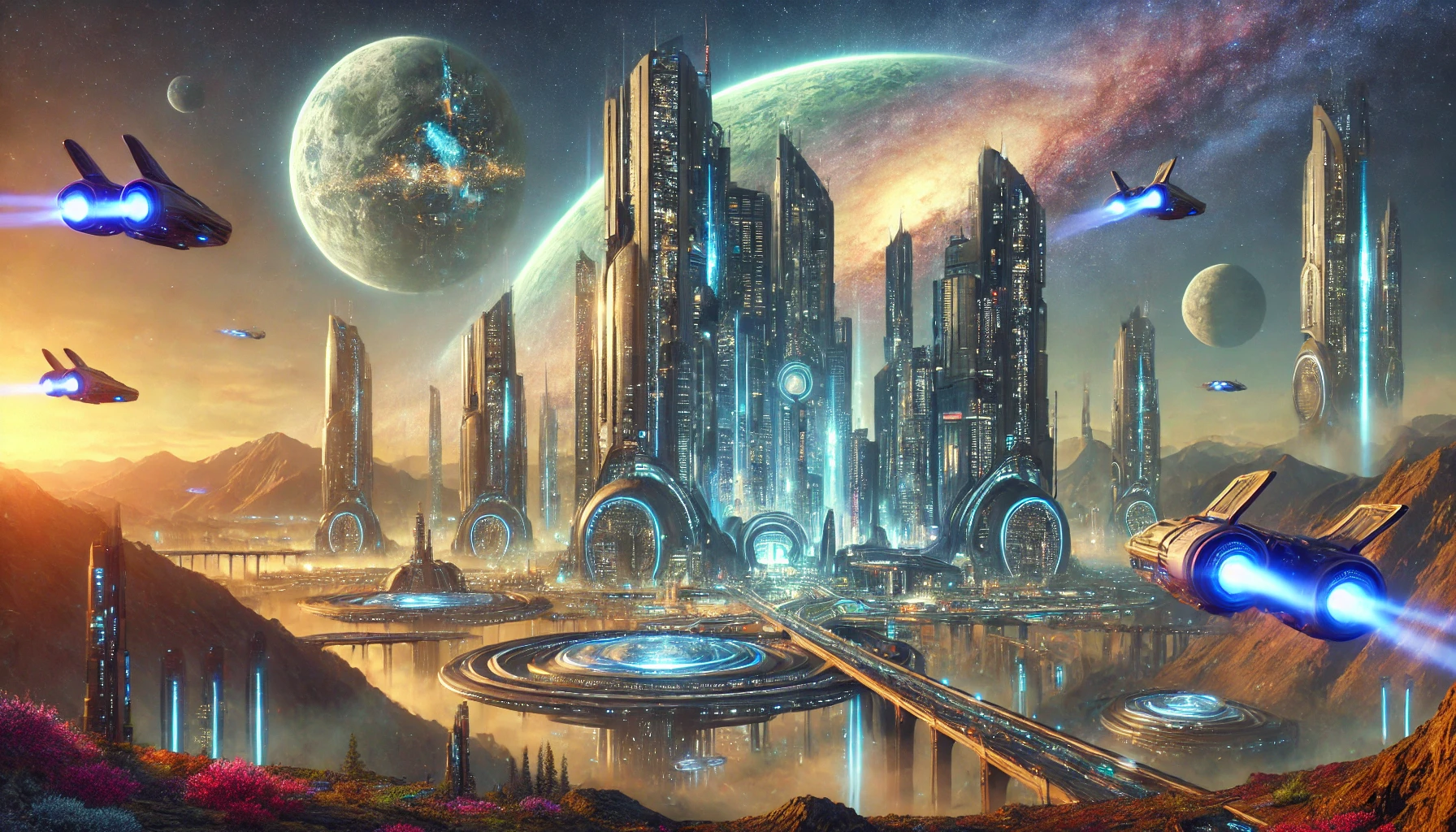